In order to grant a Lambda function access to Secrets Manager, we have to attach an IAM policy to the function’s execution role. The policy should grant permissions for all the Actions
the function needs to perform on the secrets.
For example, the following policy grants permissions for the most commonly used secrets manager actions on a specific secret.
The policy applies to a specific secret, therefore make sure to replace the YOUR_SECRET_ARN placeholder in the Resource element with the secret's ARN. You can specify multiple values if the lambda function needs access to multiple secrets.
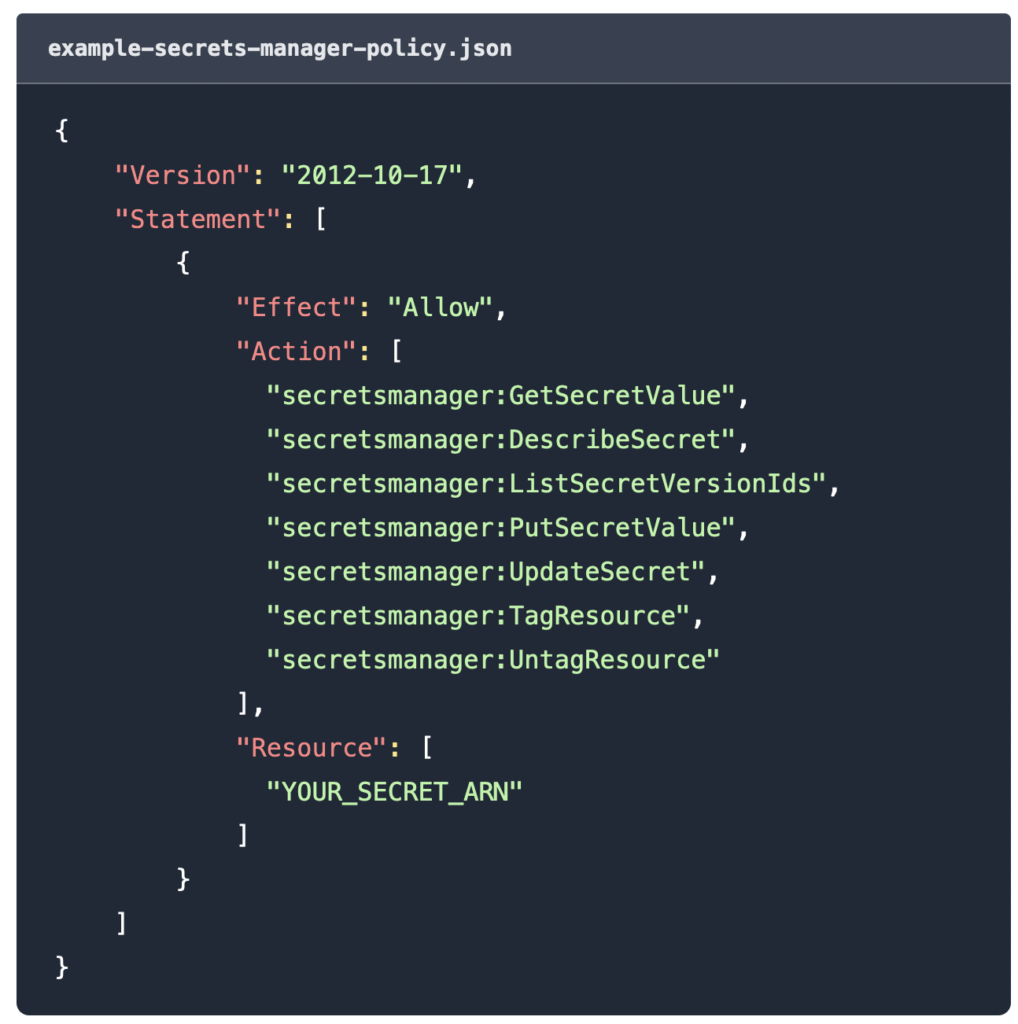
If your lambda function only needs to read a secret, you only need the secretsmanager:GetSecretValue
action.
The actions your lambda function needs to perform on the secrets are use case specific.
You could set "secretsmanager:*" for the Action element in the policy to grant full secrets manager access to the lambda function. However, it's best practice to grant an entity the least permissions that get the job done.
You can view a full list of the secrets manager Actions
in the Secrets Manager Actions table.
There is a Description
column that explains what each action does.
To attach a policy to the lambda function’s execution role:
- Open the AWS Lambda console and click on your function’s name
- Click on the
Configuration
tab and then clickPermissions
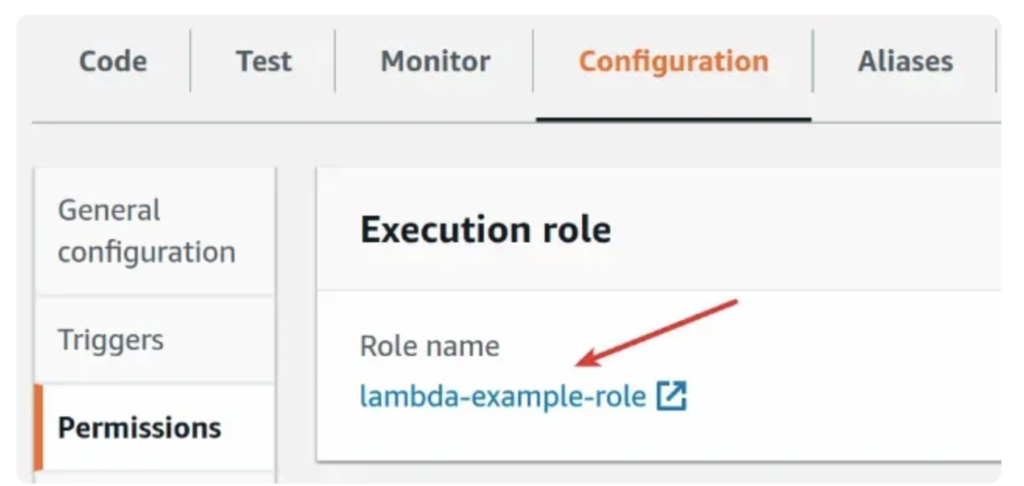
- Click on the function’s role
- Click on
Add permissions
and then clickCreate inline policy
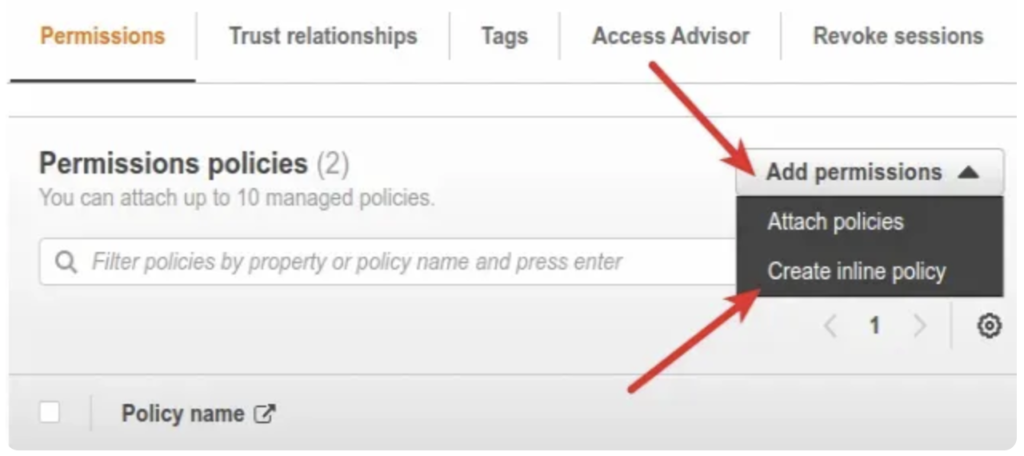
- In the
JSON
editor, paste the following policy.
Replace the YOUR_SECRET_ARN placeholder and adjust the Actions your lambda function needs to execute.
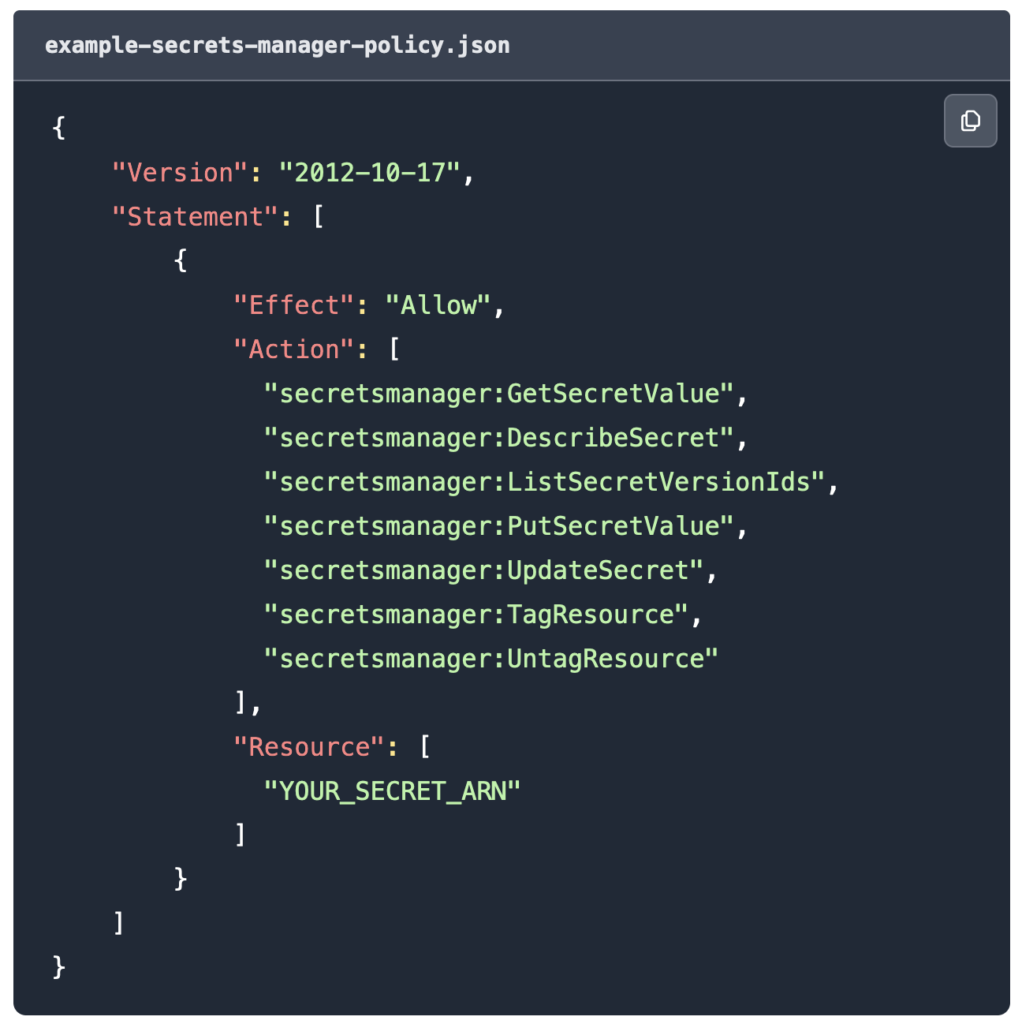
- Click
Review Policy
and give your policy a name, then clickCreate policy
At this point, the lambda function’s role has been extended with a policy that grants access to some secrets manager actions on a specific secret.
It can take up to a minute until the IAM changes have been propagated and the policy is in effect.
lambda typescript sample
import { MongoClient } from 'mongodb'; import { Axios } from 'axios'; import AWS from 'aws-sdk'; import { SecretsManagerClient, GetSecretValueCommand, } from "@aws-sdk/client-secrets-manager"; const secret_name = "cs-xxxxxx"; const client = new SecretsManagerClient({ region: "ap-northeast-1", }); export const handler = async (event) => { let response; try { response = await client.send( new GetSecretValueCommand({ SecretId: secret_name, VersionStage: "AWSCURRENT", // VersionStage defaults to AWSCURRENT if unspecified }) ); } catch (error) { // For a list of exceptions thrown, see // https://docs.aws.amazon.com/secretsmanager/latest/apireference/API_GetSecretValue.html throw error; } const secret = response.SecretString; console.log('******lambda tets start******'); console.log(event); console.log('Axios:' + Axios.toString); console.log('MongoClient:' + MongoClient.name); console.log('S3:' + AWS.S3.toString); console.log('******lambda test end******'); };