有时我们需要在IOS程序中,监听音量按下的动作,可以用下面的方法。
- 在后台模式中,打开Audio开关
只有打开这个开关,才能在锁屏模式下,监听到音量按钮
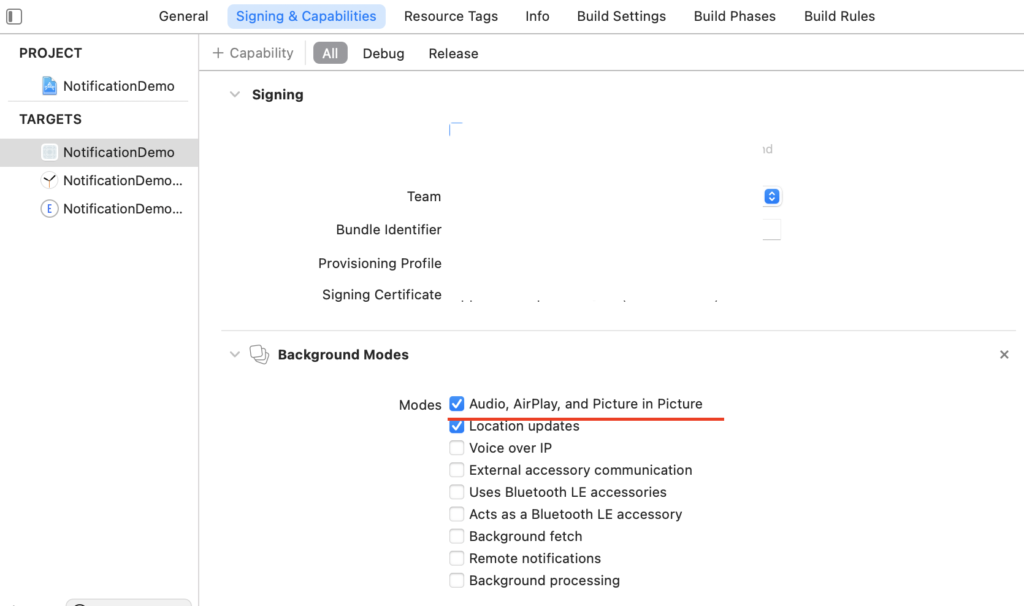
- SceneDelegate.swift 代码
import UIKit import SwiftUI import AudioToolbox class SceneDelegate: UIResponder, UIWindowSceneDelegate { var window: UIWindow? func scene(_ scene: UIScene, willConnectTo session: UISceneSession, options connectionOptions: UIScene.ConnectionOptions) { // Use this method to optionally configure and attach the UIWindow `window` to the provided UIWindowScene `scene`. // If using a storyboard, the `window` property will automatically be initialized and attached to the scene. // This delegate does not imply the connecting scene or session are new (see `application:configurationForConnectingSceneSession` instead). // Create the SwiftUI view that provides the window contents. let contentView = ContentView() // Use a UIHostingController as window root view controller. if let windowScene = scene as? UIWindowScene { let window = UIWindow(windowScene: windowScene) window.rootViewController = UIHostingController(rootView: contentView) self.window = window window.makeKeyAndVisible() } // 这里面监听音量按钮 NotificationCenter.default.addObserver(self, selector: #selector(self.changeVolumSlider), name: NSNotification.Name(rawValue: "AVSystemController_SystemVolumeDidChangeNotification"), object: nil) UIApplication.shared.beginReceivingRemoteControlEvents() } // 当音量按钮按下时,会触发下面的方法 @objc func changeVolumSlider(notifi: NSNotification) { let reason = (notifi.userInfo?["AVSystemController_AudioVolumeChangeReasonNotificationParameter"] as? String) ?? "" if reason == "ExplicitVolumeChange" { for checkPointInfo in checkPointInfoCommonList where checkPointInfo.noticeStatus == .fire { checkPointInfo.noticeStatus = .stop checkPointInfo.stopAlert() } } } func sceneDidDisconnect(_ scene: UIScene) { // Called as the scene is being released by the system. // This occurs shortly after the scene enters the background, or when its session is discarded. // Release any resources associated with this scene that can be re-created the next time the scene connects. // The scene may re-connect later, as its session was not necessarily discarded (see `application:didDiscardSceneSessions` instead). } func sceneDidBecomeActive(_ scene: UIScene) { // Called when the scene has moved from an inactive state to an active state. // Use this method to restart any tasks that were paused (or not yet started) when the scene was inactive. } func sceneWillResignActive(_ scene: UIScene) { // Called when the scene will move from an active state to an inactive state. // This may occur due to temporary interruptions (ex. an incoming phone call). } func sceneWillEnterForeground(_ scene: UIScene) { // Called as the scene transitions from the background to the foreground. // Use this method to undo the changes made on entering the background. } func sceneDidEnterBackground(_ scene: UIScene) { // Called as the scene transitions from the foreground to the background. // Use this method to save data, release shared resources, and store enough scene-specific state information // to restore the scene back to its current state. } }
- 需要特别注意,只有在音频文件播放时,才能在锁屏状态下控制音量。可以录制一个音频文件,循环播放。
AudioPlayer import Foundation import AVFoundation class AudioPlayer: NSObject, AVAudioPlayerDelegate { static let instance = AudioPlayer() static var audioPlayer: AVAudioPlayer = { return AVAudioPlayer() }() func initAudioPlayer(songTitle: String) { let songTitle = songTitle let path = Bundle.main.path(forResource: songTitle, ofType: nil, inDirectory: "music")! let url = URL(fileURLWithPath: path) do { try AVAudioSession.sharedInstance().setCategory(AVAudioSession.Category.playback) AudioPlayer.audioPlayer = try AVAudioPlayer(contentsOf: url) AudioPlayer.audioPlayer.delegate = self } catch { } } func stop() { AudioPlayer.audioPlayer.stop() } func pause() { AudioPlayer.audioPlayer.pause() } func play() { AudioPlayer.audioPlayer.play() } func currentTime() -> Int { return Int(AudioPlayer.audioPlayer.currentTime) } func getTotalTime() -> Int { return Int(AudioPlayer.audioPlayer.duration) } func audioPlayerDidFinishPlaying(_ player: AVAudioPlayer, successfully flag: Bool) { } } AudioPlayer.instance.initAudioPlayer(songTitle: "notice.mp3") AudioPlayer.instance.play()