Spring Cloud Gateway是由spring官方基于Spring5.0、Spring Boot2.x、Project Reactor 等技术开发的 网关,目的是代替原先版本中的Spring Cloud Netfilx Zuul。
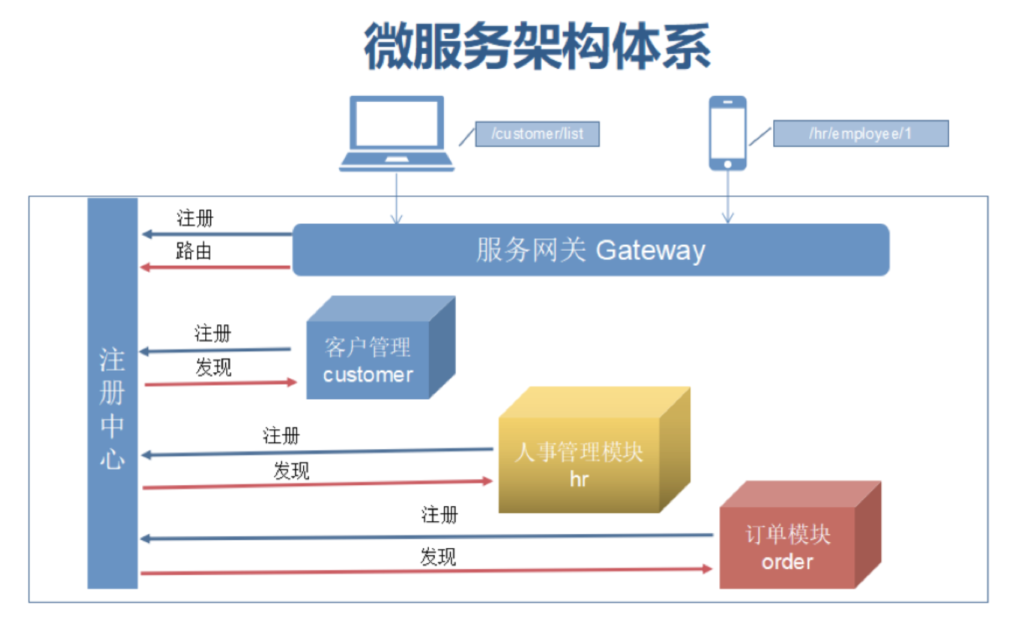
Spring Cloud Gateway 工作流程:
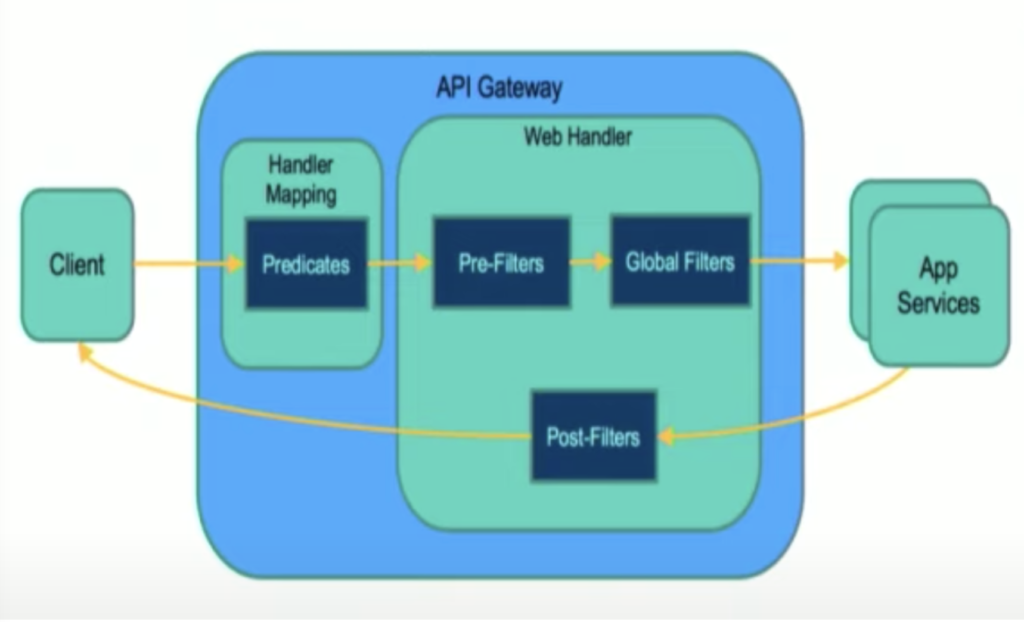
Gateway网关特性
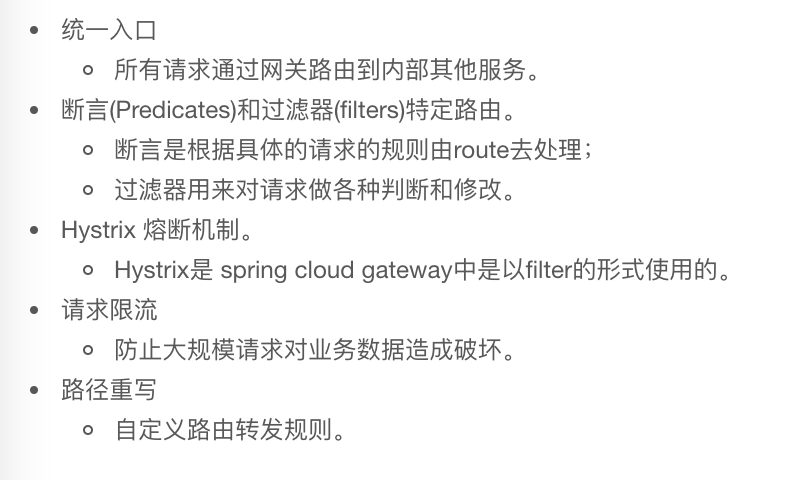
断言(Predicates)
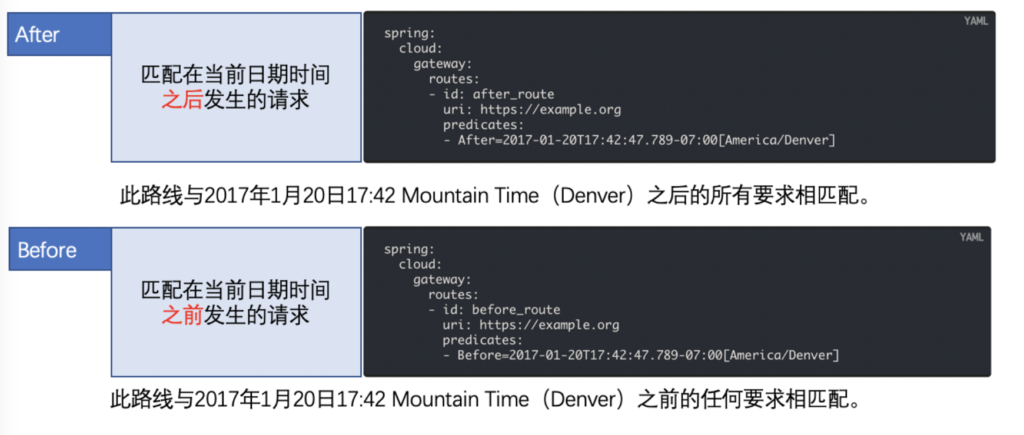
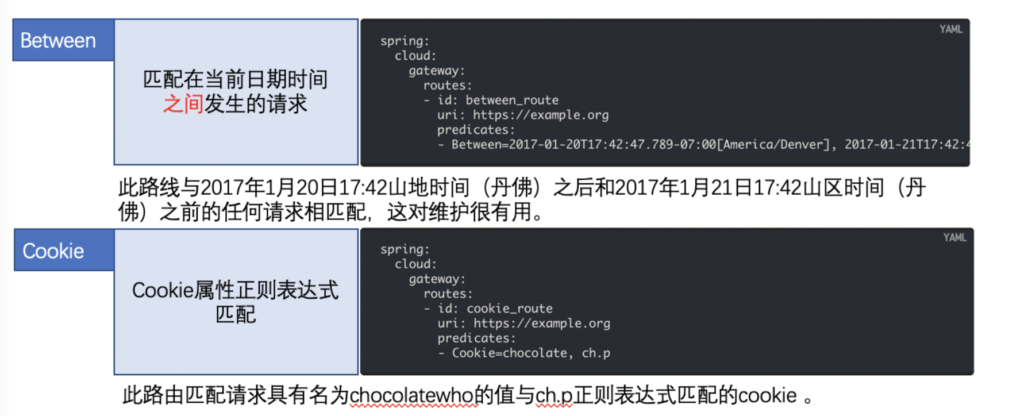
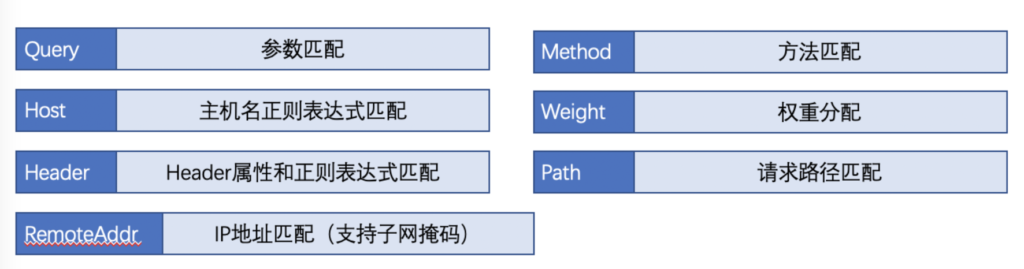
过滤器(Filter)
路由过滤器允许以某种方式修改传入的HTTP请求或传出的HTTP响应。路径过滤器的范围限定为特定路径。Spring Cloud Gateway包含许多内置的GatewayFilter工厂。
GlobalFilter 全局过滤器
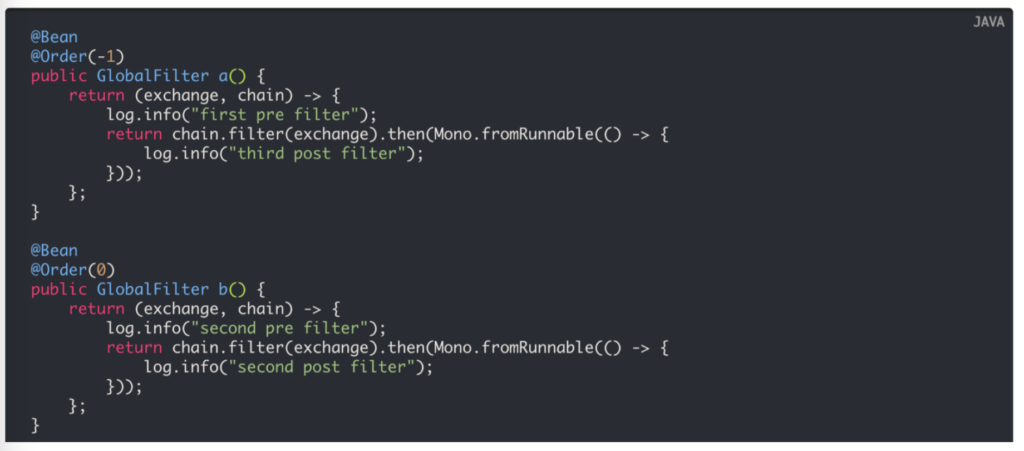
CORS跨域处理
例子:对于所有GET请求的路径,将允许来自docs.spring.io的请求的CORS请求。
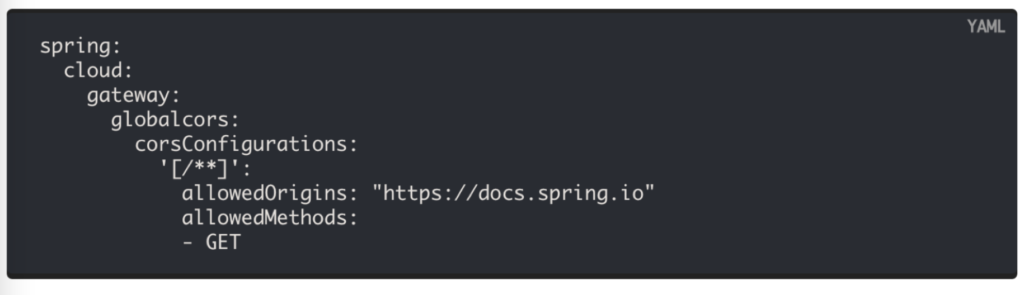
Gateway API
支持通过接口动态调整网关策略。 /actuator/gateway/refresh POST 刷新路由缓存 /actuator/gateway/routes GET 查询路由 /actuator/gateway/globalfilters GET 查询全局过滤器 /actuator/gateway/routefilters GET 查询过滤器 /actuator/gateway/routes/{id} GET、POST、DELETE 查询指定路由信息
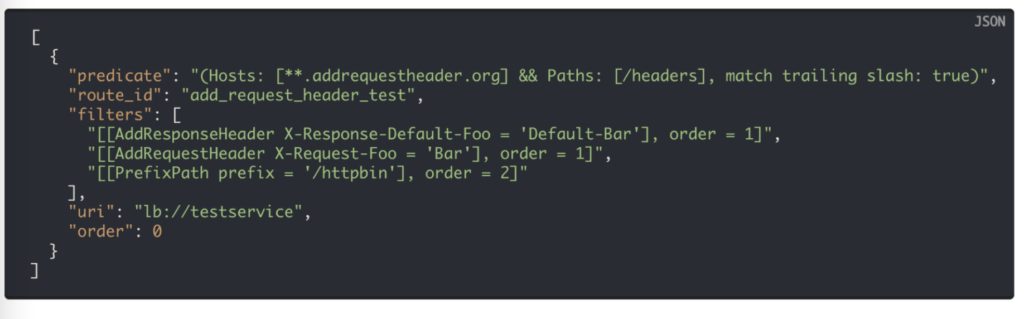
springGateWay网关搭建
- 第1步:创建一个 api-gateway 的模块,导入相关依赖
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <parent> <artifactId>springcloud-alibaba</artifactId> <groupId>com.itheima</groupId> <version>1.0-SNAPSHOT</version> </parent> <modelVersion>4.0.0</modelVersion> <artifactId>api-gateway</artifactId> <dependencies> <!--gateway网关--> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-gateway</artifactId> </dependency> </dependencies> </project>
- 第2步: 创建主类
@SpringBootApplication public class GatewayApplication { public static void main(String[] args) { SpringApplication.run(GatewayApplication.class, args); } }
- 第3步: 添加配置文件
server: port: 7000 spring: application: name: api-gateway cloud: gateway: routes: # 路由数组[路由 就是指定当请求满足什么条件的时候转到哪个微服务] - id: product_route # 当前路由的标识, 要求唯一 uri: http://localhost:8081 # 请求要转发到的地址 order: 1 # 路由的优先级,数字越小级别越高 predicates: # 断言(就是路由转发要满足的条件) - Path=/product-serv/** # 当请求路径满足Path指定的规则时,才进行路由转发 filters: # 过滤器,请求在传递过程中可以通过过滤器对其进行一定的修改 - StripPrefix=1 # 转发之前去掉1层路径
- 第4步: 启动项目, 并通过网关去访问微服务
修改成从配置中心获取路由表
- 第1步:加入nacos依赖
<!--nacos客户端--> <dependency> <groupId>com.alibaba.cloud</groupId> <artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId> </dependency>
- 第2步:在主类上添加注解
@SpringBootApplication @EnableDiscoveryClient public class ApiGatewayApplication { public static void main(String[] args) { SpringApplication.run(ApiGatewayApplication.class, args); } }
- 第3步:修改配置文件
server: port: 7000 spring: application: name: api-gateway cloud: nacos: discovery: server-addr: 127.0.0.1:8848 gateway: discovery: locator: enabled: true # 让gateway可以发现nacos中的微服务 routes: - id: product_route uri: lb://service-product # lb指的是从nacos中按照名称获取微服务,并遵循负载均 衡策略 predicates: - Path=/product-serv/** filters: - StripPrefix=1
- 第4步:测试