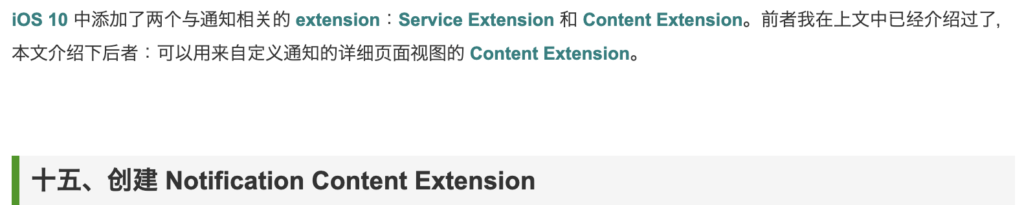
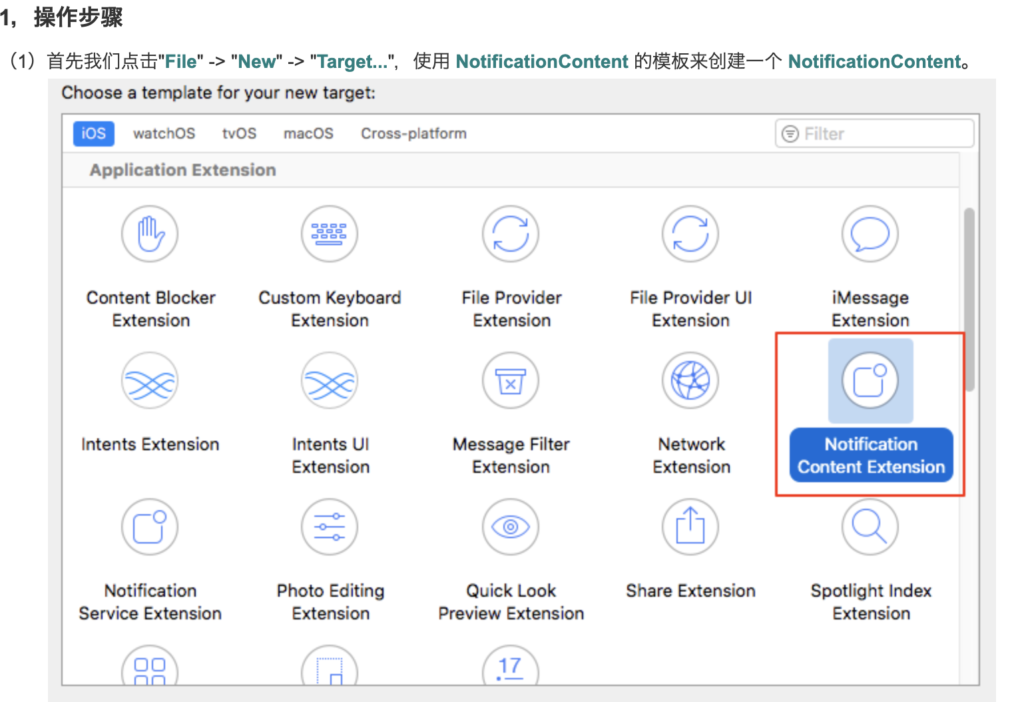
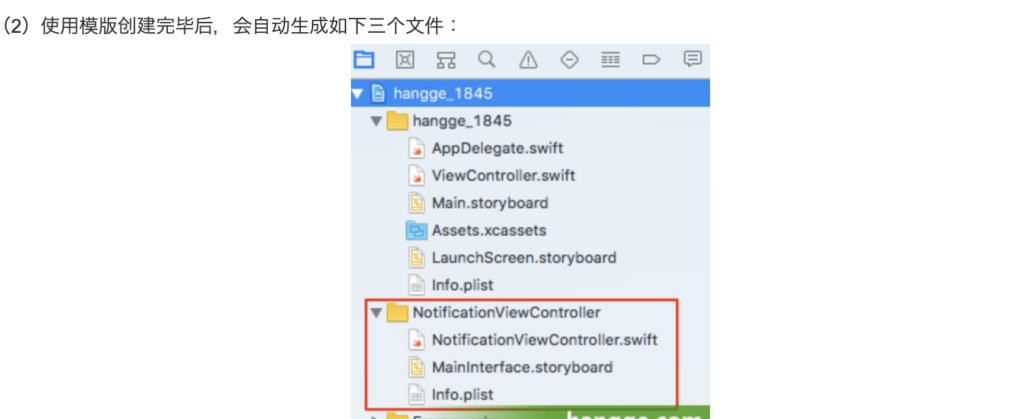

import UIKit import UserNotifications import UserNotificationsUI class NotificationViewController: UIViewController, UNNotificationContentExtension { @IBOutlet var label: UILabel? override func viewDidLoad() { super.viewDidLoad() } func didReceive(_ notification: UNNotification) { self.label?.text = notification.request.content.body } }
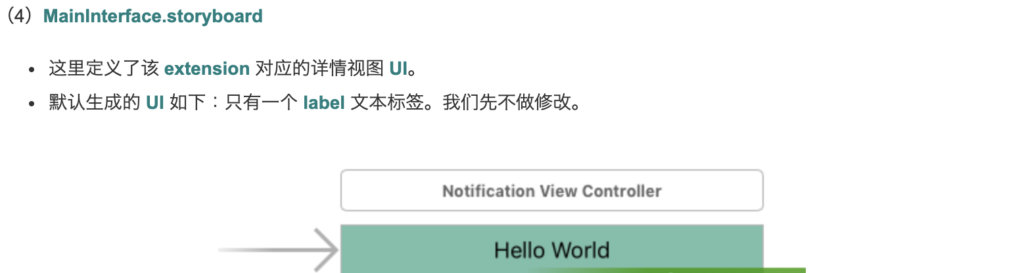
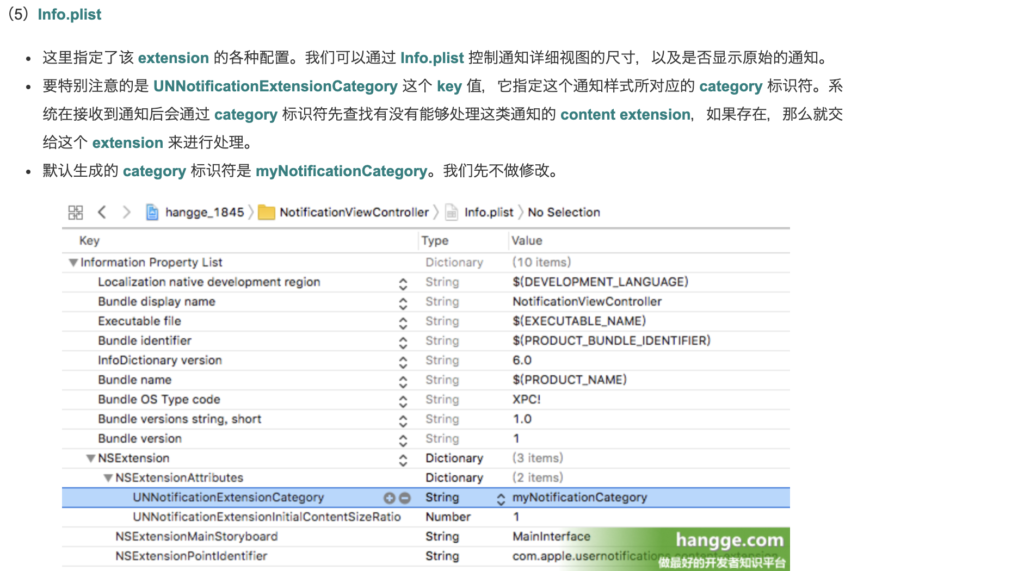

import UIKit import UserNotifications class ViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() //设置推送内容 let content = UNMutableNotificationContent() content.title = "hangge.com" content.body = "做最好的开发者知识平台" //设置category标识符 content.categoryIdentifier = "myNotificationCategory" //设置通知触发器 let trigger = UNTimeIntervalNotificationTrigger(timeInterval: 5, repeats: false) //设置请求标识符 let requestIdentifier = "com.hangge.testNotification" //设置一个通知请求 let request = UNNotificationRequest(identifier: requestIdentifier, content: content, trigger: trigger) //将通知请求添加到发送中心 UNUserNotificationCenter.current().add(request) { error in if error == nil { print("Time Interval Notification scheduled: \(requestIdentifier)") } } } override func didReceiveMemoryWarning() { super.didReceiveMemoryWarning() } }
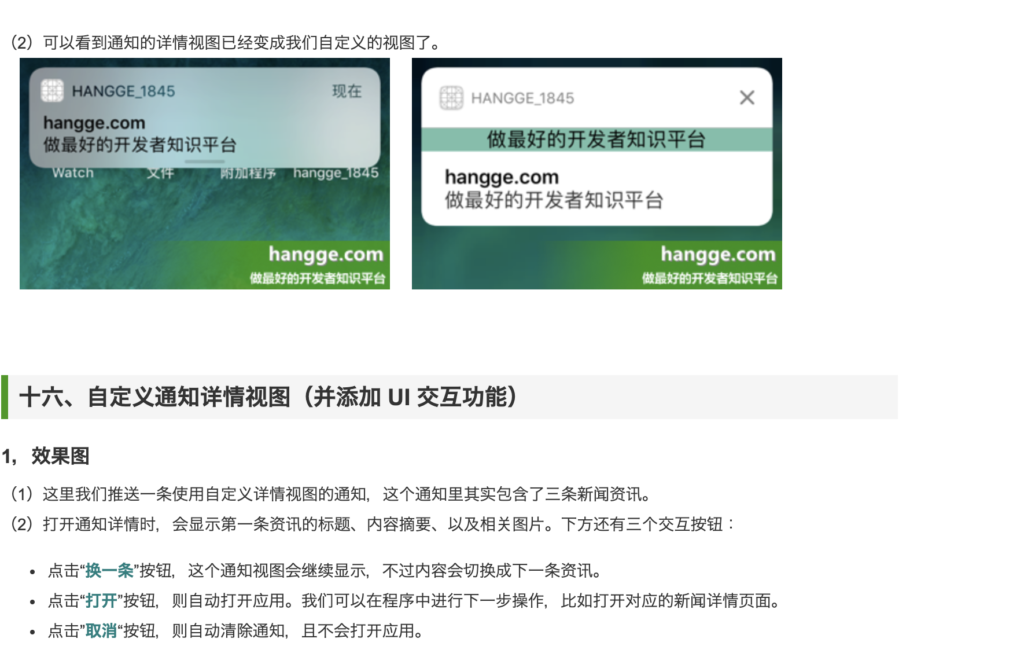
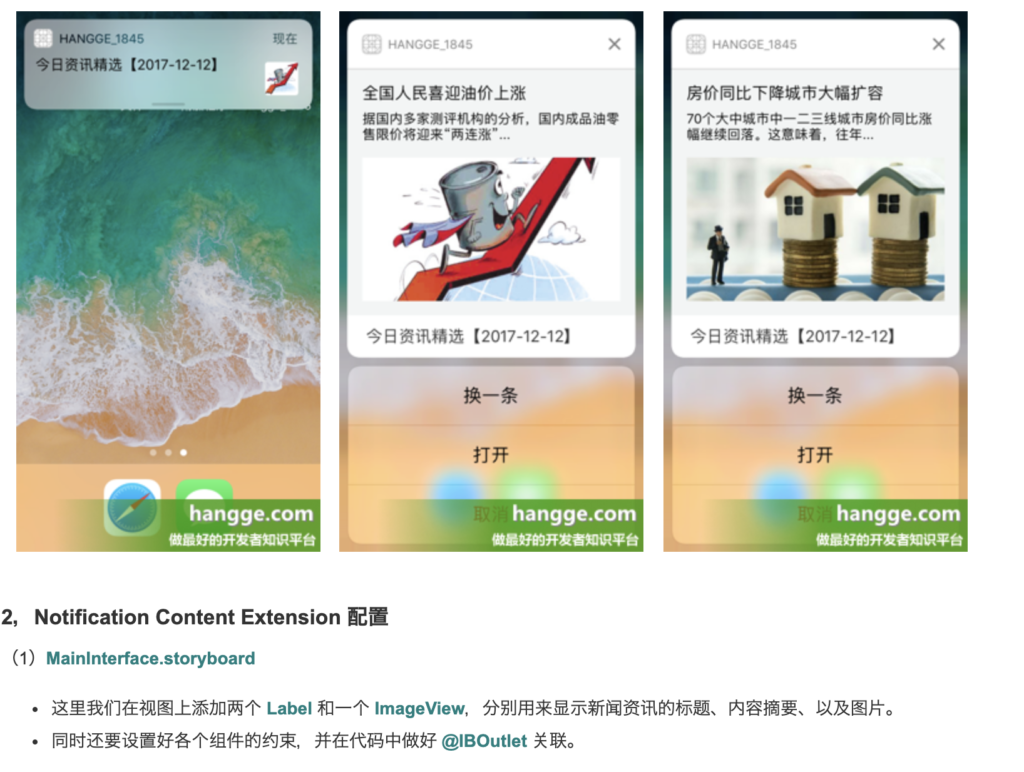
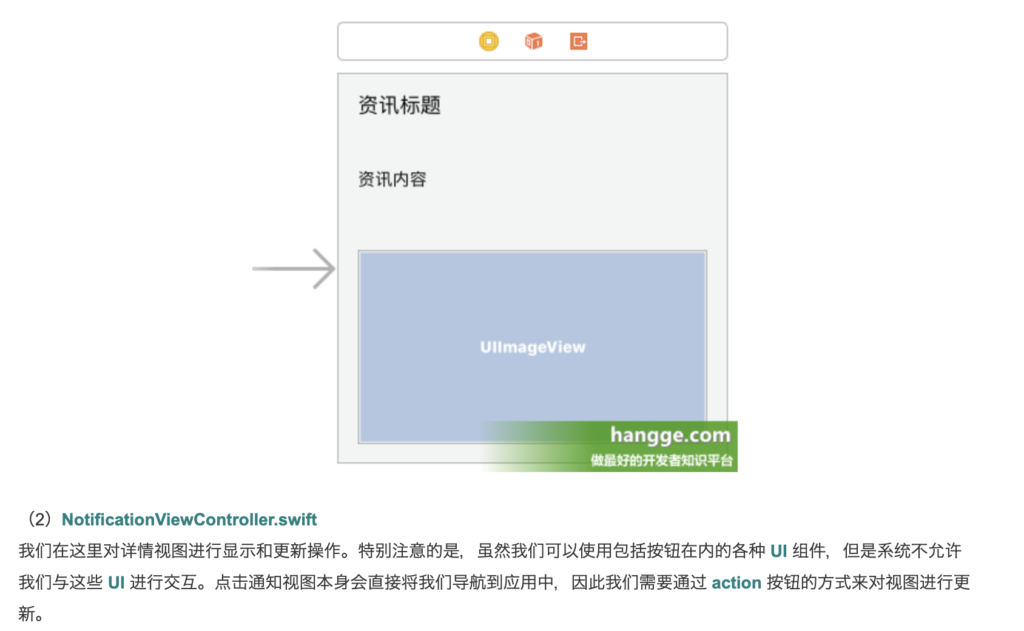
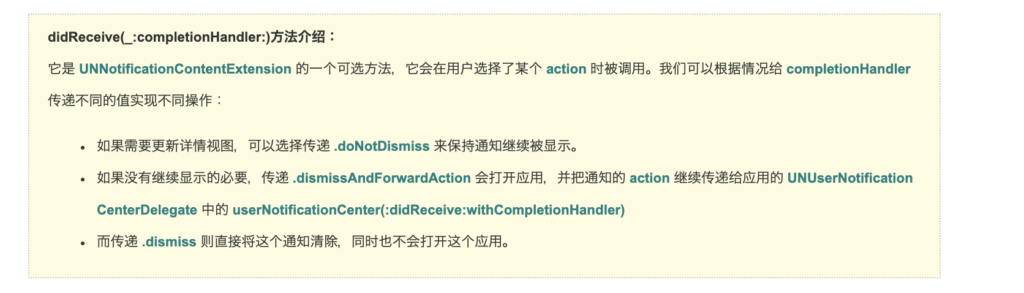
import UIKit import UserNotifications import UserNotificationsUI //资讯条目 struct NewsItem { let title: String let abstract: String let url: URL } class NotificationViewController: UIViewController, UNNotificationContentExtension { //显示资讯标题 @IBOutlet var titleLabel: UILabel! //显示资讯内容摘要 @IBOutlet weak var abstractLabel: UILabel! //显示资讯图片 @IBOutlet weak var imageView: UIImageView! //当前显示的资讯索引 private var index: Int = 0 //所有资讯条目 var items: [NewsItem] = [] override func viewDidLoad() { super.viewDidLoad() } //收到通知 func didReceive(_ notification: UNNotification) { //处理资讯条目 let content = notification.request.content if let news = content.userInfo["news"] as? [[String: String]] { for i in 0..<news.count { let title = news[i]["title"] ?? "" let abstract = news[i]["abstract"] ?? "" let url = content.attachments[i].url let presentItem = NewsItem(title: title, abstract: abstract, url: url) self.items.append(presentItem) } } //显示第一条资讯 updateNews(index: 0) } //更新显示的资讯内容 private func updateNews(index: Int) { let item = items[index] //更新标题和内容摘要 self.titleLabel!.text = item.title self.abstractLabel.text = item.abstract //更新图片 if item.url.startAccessingSecurityScopedResource() { self.imageView.image = UIImage(contentsOfFile: item.url.path) item.url.stopAccessingSecurityScopedResource() } self.index = index } //Action按钮点击响应 func didReceive(_ response: UNNotificationResponse, completionHandler completion: @escaping (UNNotificationContentExtensionResponseOption) -> Void) { if response.actionIdentifier == "change" { //切换下一条资讯 let nextIndex = (index + 1) % items.count updateNews(index: nextIndex) //保持通知继续被显示 completion(.doNotDismiss) } else if response.actionIdentifier == "open" { //取消这个通知并继续传递Action completion(.dismissAndForwardAction) } else if response.actionIdentifier == "dismiss" { //直接取消这个通知 completion(.dismiss) } else { //取消这个通知并继续传递Action completion(.dismissAndForwardAction) } } }
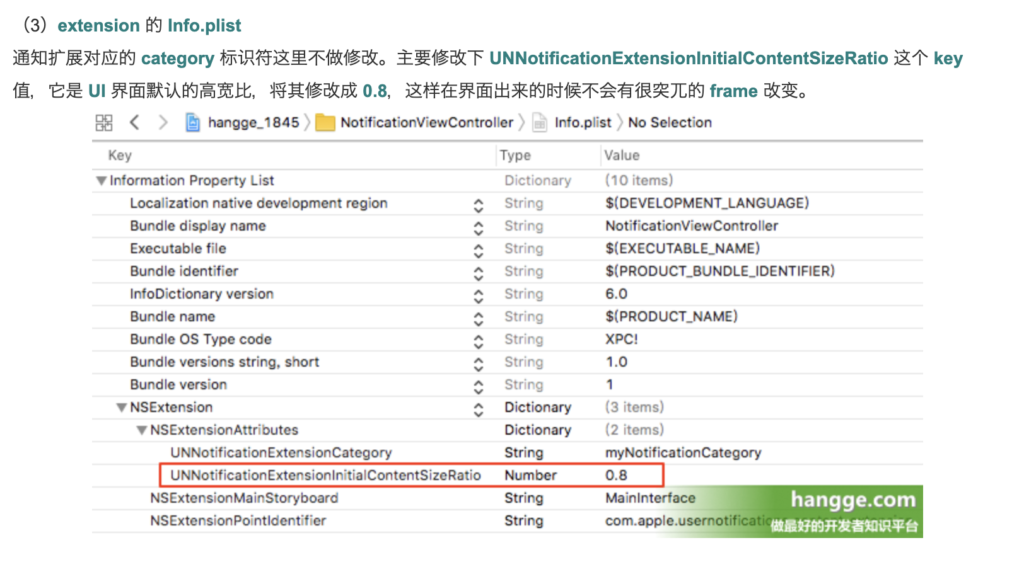

import UIKit import UserNotifications @UIApplicationMain class AppDelegate: UIResponder, UIApplicationDelegate { var window: UIWindow? func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool { UNUserNotificationCenter.current() .requestAuthorization(options: [.alert, .sound, .badge]) { (accepted, error) in if !accepted { print("用户不允许消息通知。") } } //注册category registerNotificationCategory() return true } func applicationWillResignActive(_ application: UIApplication) { } func applicationDidEnterBackground(_ application: UIApplication) { } func applicationWillEnterForeground(_ application: UIApplication) { } func applicationDidBecomeActive(_ application: UIApplication) { } func applicationWillTerminate(_ application: UIApplication) { } //注册一个category private func registerNotificationCategory() { let newsCategory: UNNotificationCategory = { //创建三个普通的按钮action let changeAction = UNNotificationAction( identifier: "change", title: "换一条", options: []) let openAction = UNNotificationAction( identifier: "open", title: "打开", options: [.foreground]) //创建普通的按钮action let cancelAction = UNNotificationAction( identifier: "cancel", title: "取消", options: [.destructive]) //创建category return UNNotificationCategory(identifier: "myNotificationCategory", actions: [changeAction, openAction, cancelAction], intentIdentifiers: [], options: []) }() //把category添加到通知中心 UNUserNotificationCenter.current().setNotificationCategories([newsCategory]) } }

import UIKit import UserNotifications class ViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() //设置推送内容 let content = UNMutableNotificationContent() content.body = "今日资讯精选【2017-12-12】" //设置通知category标识符 content.categoryIdentifier = "myNotificationCategory" //设置通知附件图片 let imageNames = ["image1", "image2", "image3"] let attachments = imageNames.flatMap { name -> UNNotificationAttachment? in if let imageURL = Bundle.main.url(forResource: name, withExtension: "png") { return try? UNNotificationAttachment(identifier: "\(name)", url: imageURL, options: nil) } return nil } content.attachments = attachments //设置通知附加信息(资讯标题和内容摘要) content.userInfo = ["news": [ ["title": "全国人民喜迎油价上涨", "abstract": "据国内多家测评机构的分析,国内成品油零售限价将迎来“两连涨”..."], ["title": "房价同比下降城市大幅扩容", "abstract": "70个大中城市中一二三线城市房价同比涨幅继续回落。这意味着,往年..."], ["title": "比特币市值再创新高", "abstract": "一项名为SegWit2X的技术取消升级,导致在本周一比特币市值蒸发多达380亿美元..."] ]] //设置通知触发器 let trigger = UNTimeIntervalNotificationTrigger(timeInterval: 5, repeats: false) //设置请求标识符 let requestIdentifier = "com.hangge.testNotification" //设置一个通知请求 let request = UNNotificationRequest(identifier: requestIdentifier, content: content, trigger: trigger) //将通知请求添加到发送中心 UNUserNotificationCenter.current().add(request) { error in if error == nil { print("Time Interval Notification scheduled: \(requestIdentifier)") } } } override func didReceiveMemoryWarning() { super.didReceiveMemoryWarning() } }