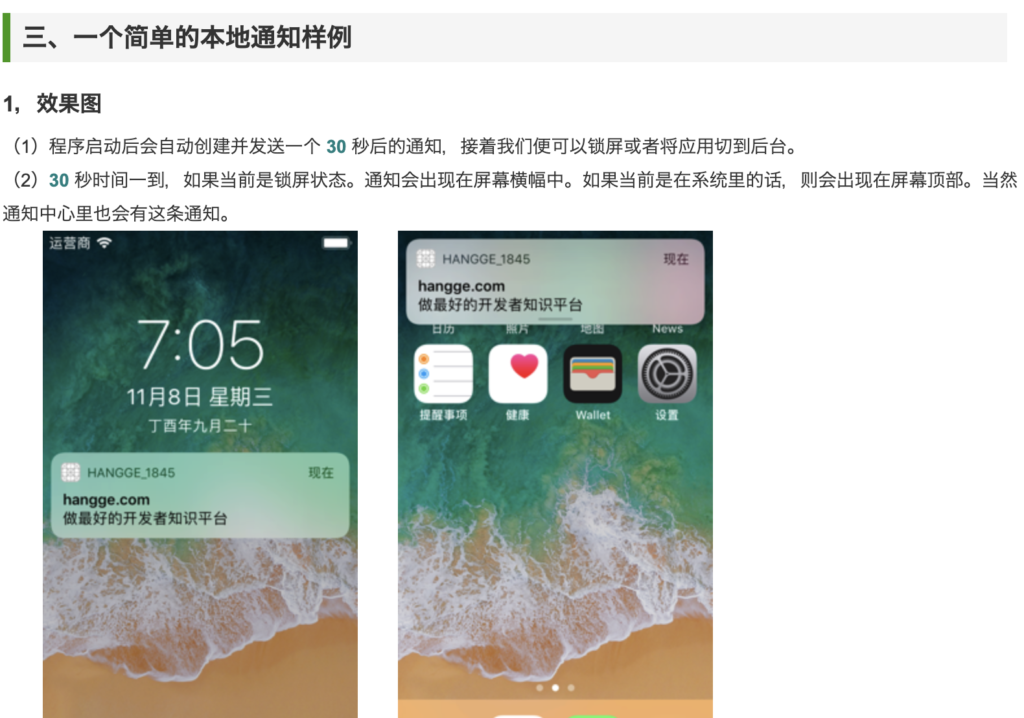

import UIKit import UserNotifications @UIApplicationMain class AppDelegate: UIResponder, UIApplicationDelegate { var window: UIWindow? func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool { //请求通知权限 UNUserNotificationCenter.current() .requestAuthorization(options: [.alert, .sound, .badge]) { (accepted, error) in if !accepted { print("用户不允许消息通知。") } } return true } func applicationWillResignActive(_ application: UIApplication) { } func applicationDidEnterBackground(_ application: UIApplication) { } func applicationWillEnterForeground(_ application: UIApplication) { } func applicationDidBecomeActive(_ application: UIApplication) { } func applicationWillTerminate(_ application: UIApplication) { } } 原文出自:www.hangge.com 转载请保留原文链接:https://www.hangge.com/blog/cache/detail_1851.html

import UIKit import UserNotifications class ViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() //设置推送内容 let content = UNMutableNotificationContent() content.title = "hangge.com" content.body = "做最好的开发者知识平台" //设置通知触发器 let trigger = UNTimeIntervalNotificationTrigger(timeInterval: 30, repeats: false) //设置请求标识符 let requestIdentifier = "com.hangge.testNotification" //设置一个通知请求 let request = UNNotificationRequest(identifier: requestIdentifier, content: content, trigger: trigger) //将通知请求添加到发送中心 UNUserNotificationCenter.current().add(request) { error in if error == nil { print("Time Interval Notification scheduled: \(requestIdentifier)") } } } override func didReceiveMemoryWarning() { super.didReceiveMemoryWarning() } }

//设置推送内容 let content = UNMutableNotificationContent() content.title = "hangge.com" content.subtitle = "航歌(二级标题)" content.body = "做最好的开发者知识平台" content.badge = 2
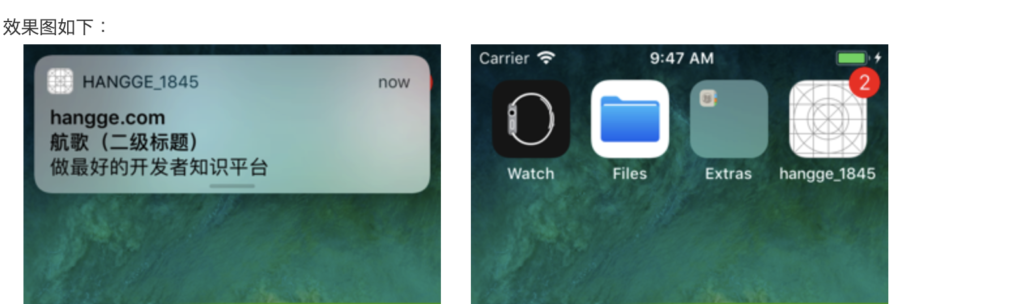
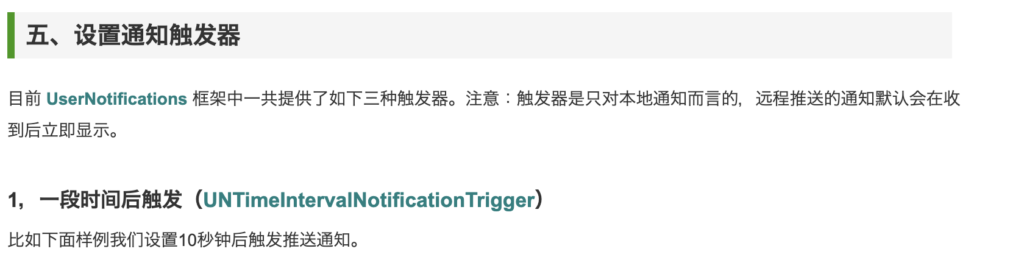
let trigger = UNTimeIntervalNotificationTrigger(timeInterval: 10, repeats: false)

var components = DateComponents() components.year = 2017 components.month = 11 components.day = 11 let trigger = UNCalendarNotificationTrigger(dateMatching: components, repeats: false)

var components = DateComponents() components.weekday = 2 //周一 components.hour = 8 //上午8点 components.second = 30 //30分 let trigger = UNCalendarNotificationTrigger(dateMatching: components, repeats: true)

let coordinate = CLLocationCoordinate2D(latitude: 52.10, longitude: 51.11) let region = CLCircularRegion(center: coordinate, radius: 200, identifier: "center") region.notifyOnEntry = true //进入此范围触发 region.notifyOnExit = false //离开此范围不触发 let trigger = UNLocationNotificationTrigger(region: region, repeats: true)