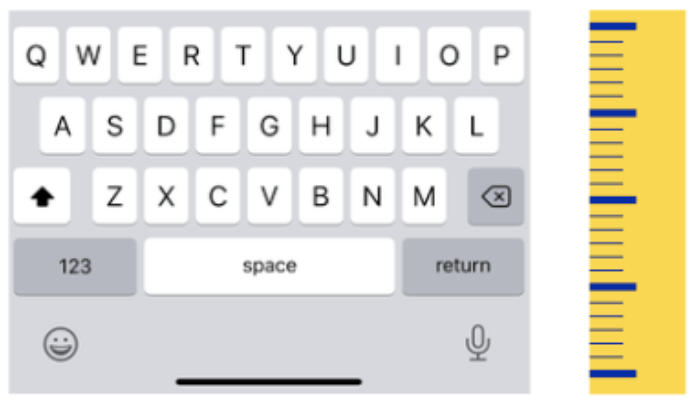
在App开发过程中,往往需要根据弹出键盘的高度来响应我们的布局,所以能否实时取得正确的键盘高度数值是一个经常会遇到的问题,我们可以通过下面的方式,来实时获取弹出键盘的高度。
Step 1: Create our keyboard height helper class
// 创建 KeyboardHeightHelper类,并且实现ObservableObject接口 class KeyboardHeightHelper: ObservableObject { // 键盘高度 @Published var keyboardHeight: CGFloat = 0 private func listenForKeyboardNotifications() { // 监听键盘弹出事件 NotificationCenter.default.addObserver(forName: UIResponder.keyboardWillShowNotification, object: nil, queue: .main) { (notification) in guard let userInfo = notification.userInfo, let keyboardRect = userInfo[UIResponder.keyboardFrameEndUserInfoKey] as? CGRect else { return } print(keyboardRect.height) self.keyboardHeight = keyboardRect.height // 通过NotificationCenter把键盘弹出的事件传递出去,SwiftUI可以通过onReceive监听接收到通知。 NotificationCenter.default.post(name: .editBegin, object: nil, userInfo: nil) } // 监听键盘关闭事件 NotificationCenter.default.addObserver(forName: UIResponder.keyboardWillHideNotification, object: nil, queue: .main) { (notification) in self.keyboardHeight = 0 // 通过NotificationCenter把键盘弹出的事件传递出去,SwiftUI可以通过onReceive监听接收到通知。 NotificationCenter.default.post(name: .editEnd, object: nil, userInfo: nil) } } init() { self.listenForKeyboardNotifications() } }
Step 2: Use the keyboardHeight value
struct keyBoardDemoView{ // 定义keyboardHeightHelper变量,设定为ObservedObject类型。 @ObservedObject var keyboardHeightHelper = KeyboardHeightHelper() @State var textFieldText = "" var body: some View { VStack { Spacer() TextField("Text field", text: $textFieldText) // 可以利用监听得到的keyboardHeight键盘高度,通过SwiftUI的offset自由的调整文本框的位置,以免被弹出键盘覆盖掉。 .offset(y: -self.keyboardHeightHelper.keyboardHeight) } } }