有时候我们需要在Swift中,播放声音,有可能是系统的声音,有可能是自定义的声音。这里需要借助AVFoundation的AVAudioPlayer。
- 下面是Sample的代码。
import Foundation import MapKit import MediaPlayer import AVFoundation import UIKit import AudioToolbox class AudioPlayer: NSObject, AVAudioPlayerDelegate { // 单例模式 static let instance = AudioPlayer() var isSoundPlaying = false static var audioPlayer: AVAudioPlayer = { return AVAudioPlayer() }() // ※播放自定义的声音,需要把声音文件拷贝到工程目录中,并且指定这个目录和声音文件的名字。 func play(songTitle: String) { let songTitle = songTitle let path = Bundle.main.path(forResource: songTitle, ofType: nil, inDirectory: "Music")! let url = URL(fileURLWithPath: path) do { try AVAudioSession.sharedInstance().setCategory(AVAudioSession.Category.playback) AudioPlayer.audioPlayer = try AVAudioPlayer(contentsOf: url) // ※AVAudioPlayerDelegate代理 AudioPlayer.audioPlayer.delegate = self AudioPlayer.audioPlayer.play() } catch { } } // ※播放系统的声音 需要知道系统声音的ID 可以去网站查询。 // ※https://iphonedev.wiki/index.php/AudioServices func play(id: UInt32) { AudioServicesPlaySystemSound(id) } func stop() { AudioPlayer.audioPlayer.stop() } func pause() { AudioPlayer.audioPlayer.pause() } func resume() { AudioPlayer.audioPlayer.play() } func currentTime() -> Int { return Int(AudioPlayer.audioPlayer.currentTime) } // ※当音频文件播放完成时,会触发这个方法。 func audioPlayerDidFinishPlaying(_ player: AVAudioPlayer, successfully flag: Bool) { AudioPlayer.audioPlayer.stop() NotificationCenter.default.post(name: .needToStop, object: nil, userInfo: nil) } }
- 自定义的声音文件需要拷贝到工程里面
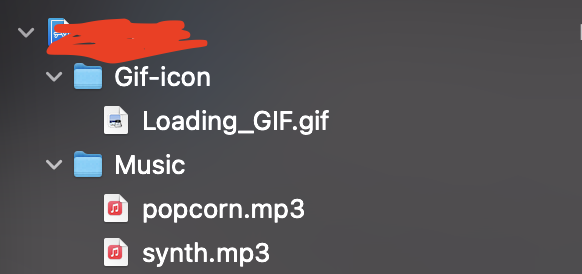
- 播放系统声音需要用id,可以从网站(https://iphonedev.wiki/index.php/AudioServices)查询声音文件的id。
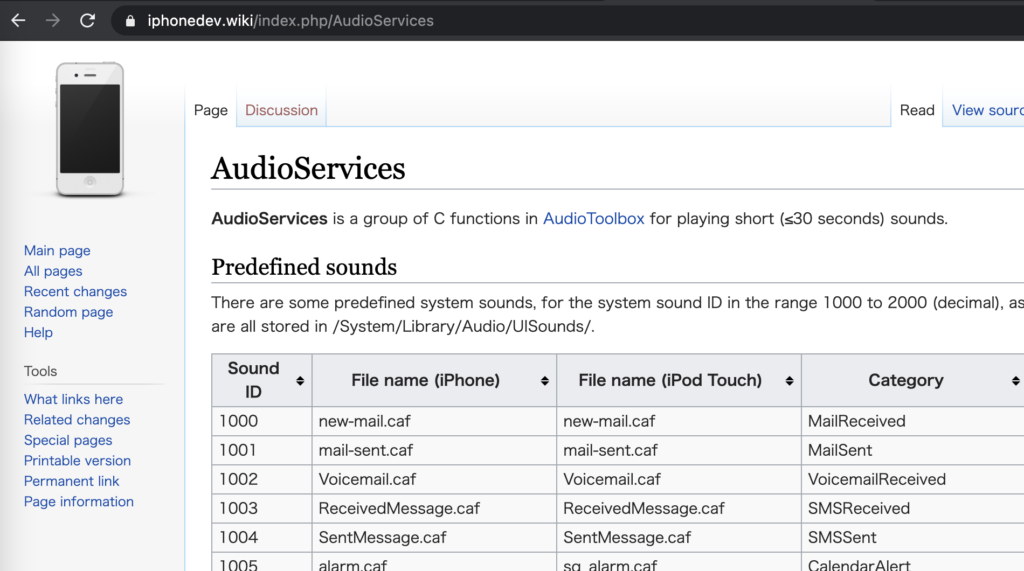
- 可以从网站(https://www.zedge.net/find/synth )下载各种声音文件。
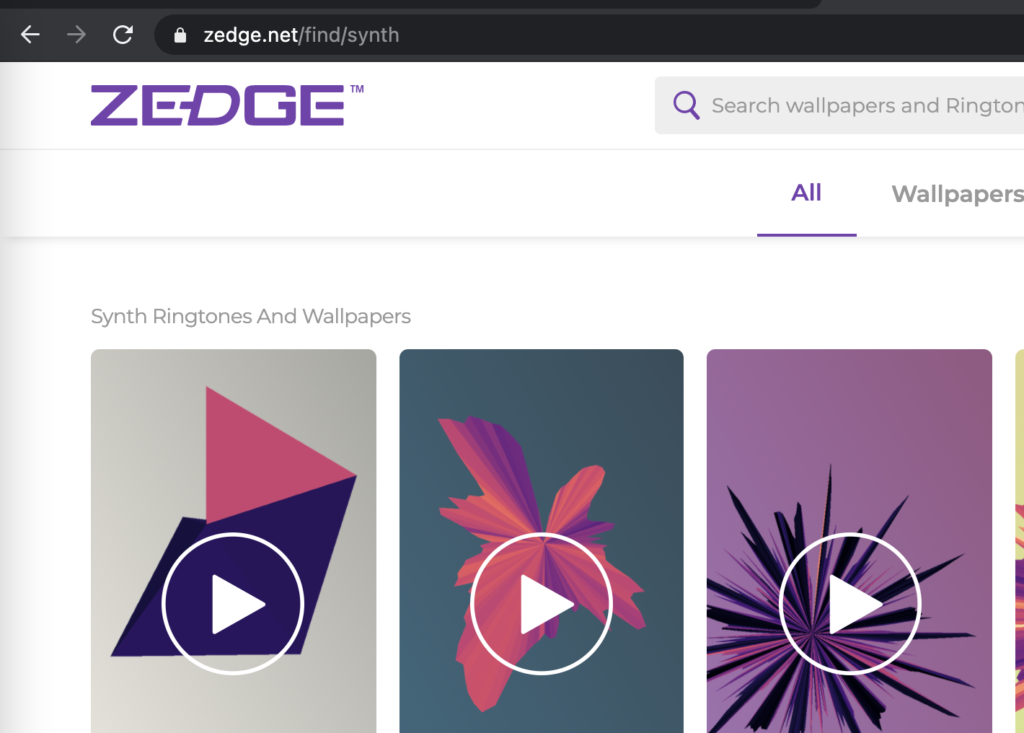