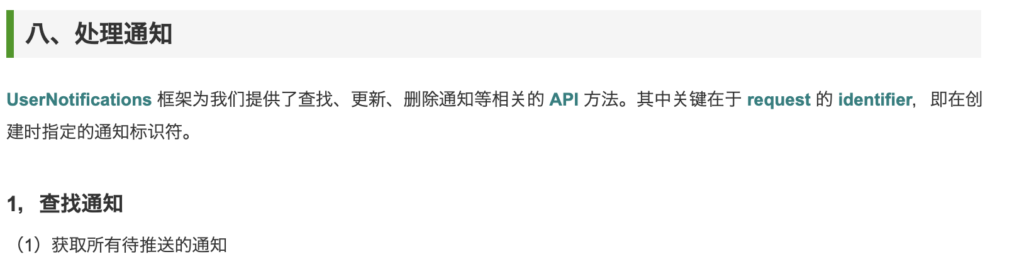
UNUserNotificationCenter.current().getPendingNotificationRequests { (requests) in //遍历所有未推送的request for request in requests { print(request) } }
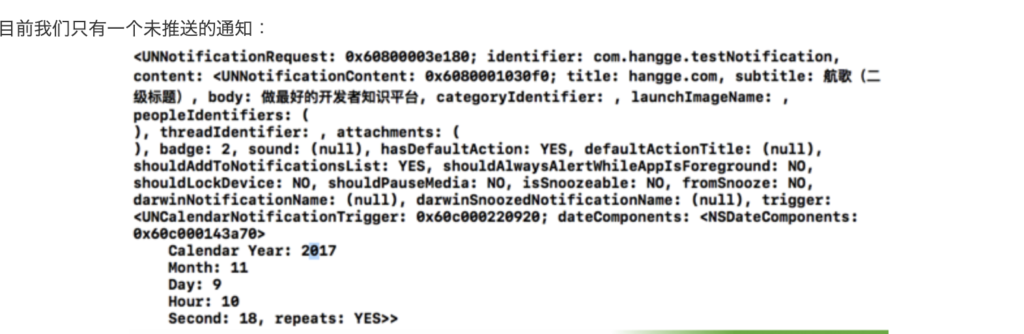

UNUserNotificationCenter.current().getDeliveredNotifications { (notifications) in //遍历所有已推送的通知 for notification in notifications { print(notification) } }

//使用同样的请求标识符来设置一个新的通知 let requestIdentifier = "com.hangge.testNotification" let request = UNNotificationRequest(identifier: requestIdentifier, content: content, trigger: trigger) //将通知请求添加到发送中心 UNUserNotificationCenter.current().add(request) { error in if error == nil { print("Time Interval Notification scheduled: \(requestIdentifier)") } }

//根据identifier来取消指定通知 let identifier = "com.hangge.testNotification" UNUserNotificationCenter.current().removePendingNotificationRequests(withIdentifiers: [identifier]) //取消全部未发送通知 UNUserNotificationCenter.current().removeAllPendingNotificationRequests()

//根据identifier来删除指定通知 let identifier = "com.hangge.testNotification" UNUserNotificationCenter.current().removeDeliveredNotifications(withIdentifiers: [identifier]) //删除全部已发送通知 UNUserNotificationCenter.current().removeAllDeliveredNotifications()

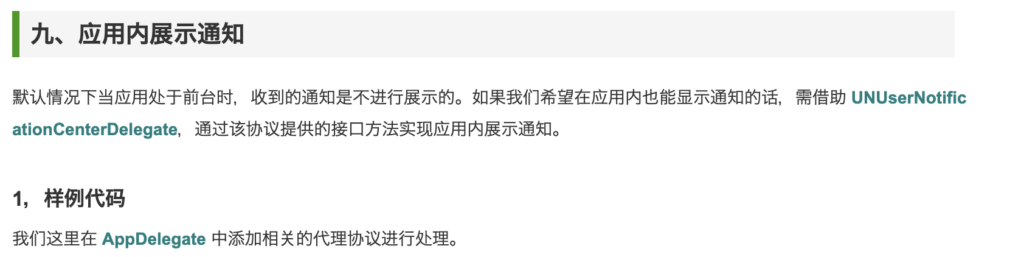
import UIKit import UserNotifications @UIApplicationMain class AppDelegate: UIResponder, UIApplicationDelegate { var window: UIWindow? let notificationHandler = NotificationHandler() func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool { //请求通知权限 UNUserNotificationCenter.current() .requestAuthorization(options: [.alert, .sound, .badge]) { (accepted, error) in if !accepted { print("用户不允许消息通知。") } } //设置通知代理 UNUserNotificationCenter.current().delegate = notificationHandler return true } func applicationWillResignActive(_ application: UIApplication) { } func applicationDidEnterBackground(_ application: UIApplication) { } func applicationWillEnterForeground(_ application: UIApplication) { } func applicationDidBecomeActive(_ application: UIApplication) { } func applicationWillTerminate(_ application: UIApplication) { } } class NotificationHandler: NSObject, UNUserNotificationCenterDelegate { //在应用内展示通知 func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) { completionHandler([.alert, .sound]) // 如果不想显示某个通知,可以直接用空 options 调用 completionHandler: // completionHandler([]) } }
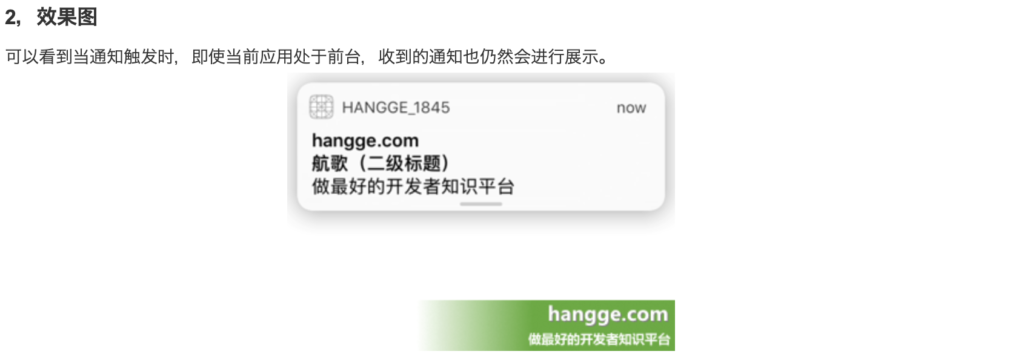
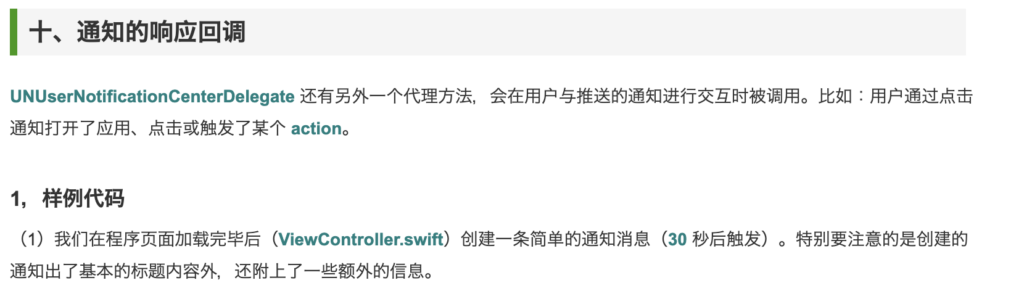
import UIKit import UserNotifications class ViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() //设置推送内容 let content = UNMutableNotificationContent() content.title = "hangge.com" content.body = "做最好的开发者知识平台" content.userInfo = ["userName": "hangge", "articleId": 10086] //设置通知触发器 let trigger = UNTimeIntervalNotificationTrigger(timeInterval: 30, repeats: false) //设置请求标识符 let requestIdentifier = "com.hangge.testNotification" //设置一个通知请求 let request = UNNotificationRequest(identifier: requestIdentifier, content: content, trigger: trigger) //将通知请求添加到发送中心 UNUserNotificationCenter.current().add(request) { error in if error == nil { print("Time Interval Notification scheduled: \(requestIdentifier)") } } } override func didReceiveMemoryWarning() { super.didReceiveMemoryWarning() } }

import UIKit import UserNotifications @UIApplicationMain class AppDelegate: UIResponder, UIApplicationDelegate { var window: UIWindow? let notificationHandler = NotificationHandler() func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool { //请求通知权限 UNUserNotificationCenter.current() .requestAuthorization(options: [.alert, .sound, .badge]) { (accepted, error) in if !accepted { print("用户不允许消息通知。") } } //设置通知代理 UNUserNotificationCenter.current().delegate = notificationHandler return true } func applicationWillResignActive(_ application: UIApplication) { } func applicationDidEnterBackground(_ application: UIApplication) { } func applicationWillEnterForeground(_ application: UIApplication) { } func applicationDidBecomeActive(_ application: UIApplication) { } func applicationWillTerminate(_ application: UIApplication) { } } class NotificationHandler: NSObject, UNUserNotificationCenterDelegate { //对通知进行响应(用户与通知进行交互时被调用) func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) { print(response.notification.request.content.title) print(response.notification.request.content.body) //获取通知附加数据 let userInfo = response.notification.request.content.userInfo print(userInfo) //完成了工作 completionHandler() } }
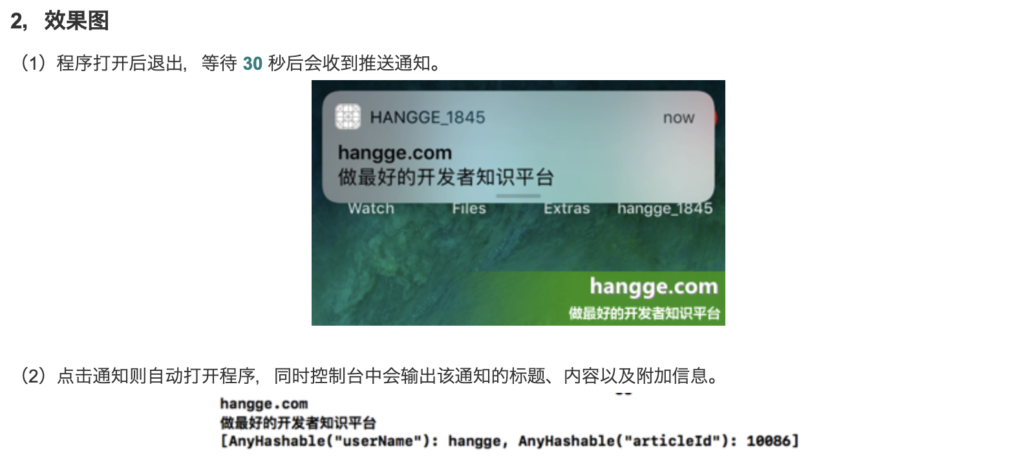